Solving the Circular Array Rotation programming exercise
How to solve the Circular Array Rotation programming exercise in Ruby?
Here's the exercise on HackerRank.
Circular Array Rotation | HackerRank
Print the elements in an array after âkâ right circular rotation operations.
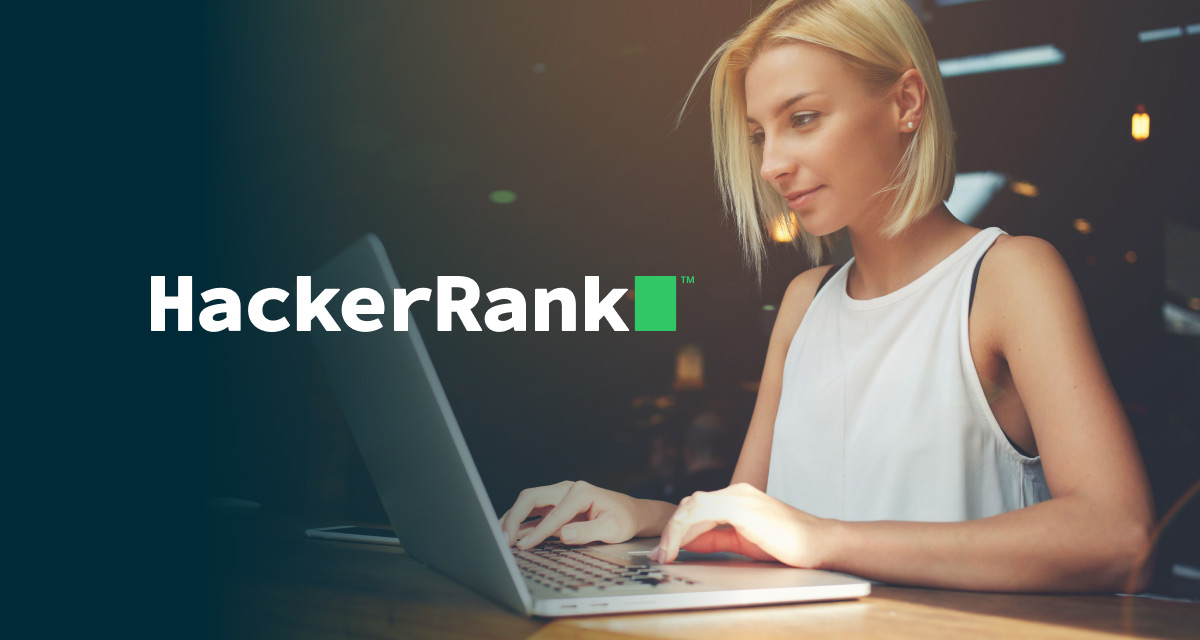
Here's my video on YouTube:
This is the initial algorithm I thought of:
Start
Input a, k and queries
enumerate from 0 to k
can be done by generating an array or use of while loop
pop last element
unshift first element
loop resulting array and return another array based on indices from queries**
End
** However, I didn't really understand immediately what value to return at the end of the method.
That's why it's always essential to know the inputs and outputs well before starting to work on any task.
Here are some snippets that might be helpful in regard to this exercise:
How to generate an array from 0 to x?
x = 10
array = Array.new(x) { |i| i }
puts array.inspect
While loop in Ruby
x = 0
while x < 5 do
puts "x is #{x}"
x += 1
end
Pop an array
Pop means to remove and return the last element in an array.
array = [1, 2, 3, 4, 5]
last_element = array.pop
puts last_element
puts array.inspect
Unshift an array
Unshift is to add an element to the first place in an array.
array = [2, 3, 4, 5]
array.unshift(1)
puts array.inspect
Loop array
array = [1, 2, 3, 4, 5]
array.each do |element|
puts element
end